How to Automatically Update Copyright Year in 2025
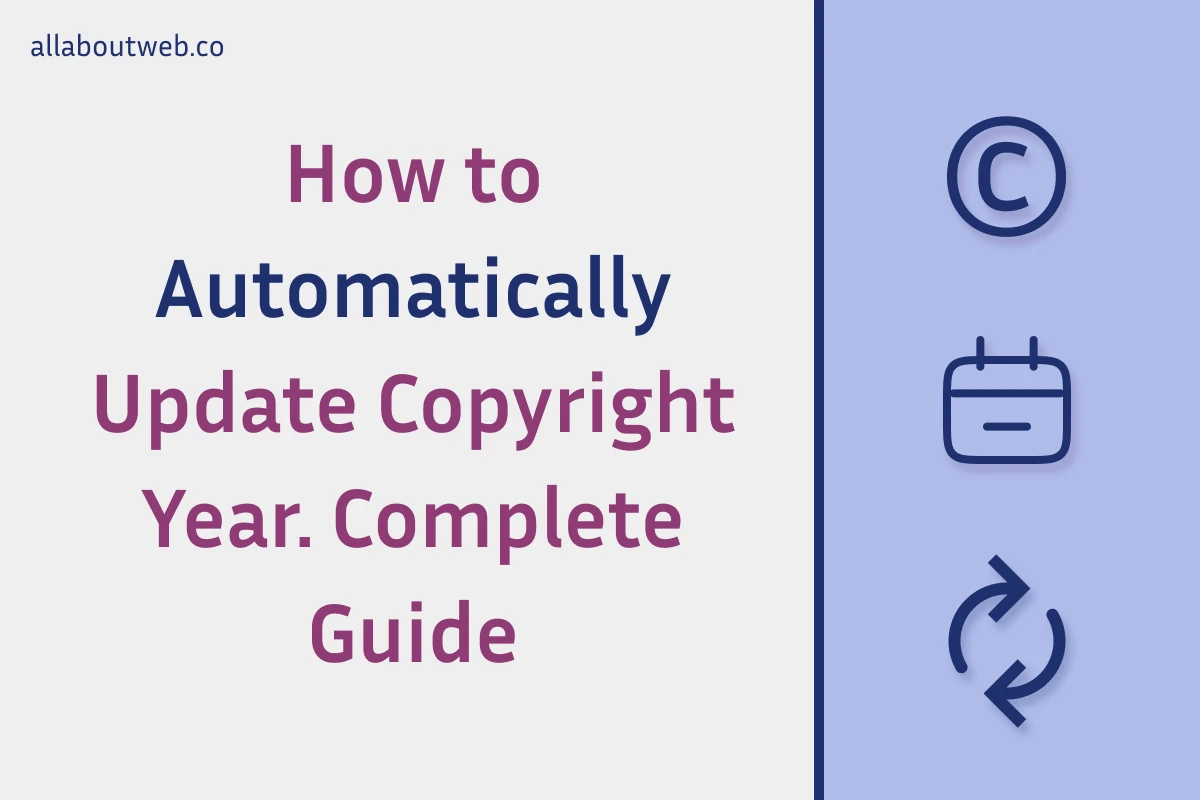
Many website owners often forget to update the copyright year, so they have it outdated until they notice it. However, it’s quite easy to set the auto update and forget about it forever.
To automatically update the copyright year, retrieve the copyright text from the copyright HTML element in the website footer. Replace the existing copyright year with the current year using the “new Date().getFullYear()” JavaScript code, and then inject the updated copyright text back into its HTML element.
Let’s dive into details and see how to do this for different scenarios.
Right before we start: this article aims to cover the topic in general. If you built your website with a constructor, you might be interested in reading more specific guides I’ve written for WordPress, Wix, Shopify, Squarespace, Webflow, Weebly and Blogger copyright. However, don’t neglect reading this one to get some helpful insights.
Does the Copyright Year Need to Be Updated Every Year?
Updating the copyright year on a website annually is a common practice but not legally required. Copyright protection is automatic upon original work creation, and the notice is more of a communication tool. While some websites automate the year update, others use a range of years to indicate when the content was created or last updated.
Check out this post to learn more about the copyright in general: Copyright Footer - Examples, HTML, Symbol, Format and More
How to Auto Update the Copyright Year with HTML and JavaScript
The Auto-Update Algorithm
Code implementation of dynamically changing copyright year may vary based on a website rendering type (e.g., Server Side Rendering, Single Page Application, Static Site Generation), a framework (e.g., React, Angular, Vue, Svelte, Next, Laravel), or a programming language.
However, the algorithm remains the same regardless of the details:
- Calculate the current year
- Get the copyright notice text
- Inject the current year value into the copyright notice text
- Render the copyright notice text
HTML Code Example of Auto Updated Current Copyright Year
Let’s see how it works for a basic scenario with pure HTML and Javascript. Nowadays, it’s a rarely used approach, modern web development utilizes a broad spectrum of frameworks and concepts. Nonetheless, it’s a good entry point to understand the basics.
<body>
<!-- Page body content HTML -->
<footer>
<!-- Footer content HTML -->
<p id="copyright">
Copyright © 2022 - 2025 allaboutweb.co
</p>
</footer>
<script>
window.addEventListener('load', onLoad);
function onLoad () {
const copyrightEl = document.getElementById('copyright');
if (copyrightEl) {
const currentYear = new Date().getFullYear();
const copyrightText = `Copyright © 2022 - ${currentYear} allaboutweb.co`;
copyrightEl.innerHTML = copyrightText;
}
}
</script>
</body>
Analyzing Code Examples and Alternative Solutions
Let’s see what we do in this code snippet to get a clear picture of how it works:
- We put
<script>...</script>
tag right before the closing</body>
tag - We define the onLoad function inside the script tag. This function manages auto updating logic:
- We select the copyright paragraph
<p id="copyright">...</p>
element by its id attribute and assign it to the copyrightEl constant variableconst copyrightEl = document.getElementById('copyright');
- We check if this element exists before modifying it
if (copyrightEl)
- We calculate the current year value and assign it to the currentYear constant variable
const currentYear = new Date().getFullYear();
- We assign a new copyright text with an injected currentYear value to the copyrightText constant variable const
copyrightText = `Copyright © 2022 - ${currentYear} allaboutweb.co`;
. Where©
is a special HTML code for copyright symbol ”©”, you can also use Decimal code©
and Hex code©
as an alternative; - We set the new text value as an inner HTML of the copyright element
copyrightEl.innerHTML = copyrightText;
- We select the copyright paragraph
- We set a listener for the load event and assign onLoad function as its handler
window.addEventListener('load', onLoad);
. As soon as a page finishes loading, the event handler executes. You can read more about it here
OK, now it’s clear!
Although does it make sense to rewrite the entire text instead of its particular piece? Well, this is a lightweight operation and there’s no big difference in implementation, so it’s just a matter of personal choice.
In any case, here’s an alternative way to do the same job:
<body>
<!-- Page body content HTML -->
<footer>
<!-- Footer content HTML -->
<p>
Copyright © 2022 - <span id="current-year">2025</span> allaboutweb.co
</p>
</footer>
<script>
window.addEventListener('load', onLoad);
function onLoad () {
const currentYearEl = document.getElementById('current-year');
if (currentYearEl) {
const currentYear = new Date().getFullYear();
currentYearEl.textContent = currentYear;
}
}
</script>
</body>
So, what’s next? How can we make this code a little bit better?
As you can see, we put the automation script right before the closing </body>
. Is it valid? Yes, but that is not considered as best practice.
What we can to is move the script into the <head />
tag. That is a bit better but having it as a separate file would be even better.
Let’s say we have the following file structure:
- root
- src
- js
- css
index.html
In the first step, we create a JS file named copyright-year-auto-update.js
and put it into the root/src/js
directory, with the file content:
window.addEventListener('load', onLoad);
function onLoad () {
const currentYearEl = document.getElementById('current-year');
if (currentYearEl) {
const currentYear = new Date().getFullYear();
currentYearEl.textContent = currentYear;
}
}
As you can see, we don’t use the <script />
tag here, because it’s not an HTML file anymore.
After that, we need to link the file to the index.html <head />
tag:
<head>
<!-- HEAD content -->
<title>How to Automatically Update Copyright Year in 2025</title>
<script src="/js/copyright-year-auto-update.js" type="text/javascript"></script>
</head>
Now, we import the script content from the /js/copyright-year-auto-update.js
file that we’ve just created, instead of mixing it up with HTML code.
How to Automatically Update Copyright Year in Popular Website Builders
Dynamic Copyright Year in WordPress
The easiest way to auto-update the copyright year in WordPress is to install the Dynamic Copyright Year plugin. You don’t need to do anything else as soon as the format of your copyright notice matches the one in the plugin description.
You can use the Auto Copyright Year Updater plugin as an alternative but it requires using the Shortcode block, which might be problematic sometimes.
If you are skeptical about installing yet another plugin, I have a solution for that, you can find it in the guide below.
Detailed guide (plugins + custom HTML): How to Automatically Update the Copyright Year in WordPress
Dynamic Copyright Year in Wix
In Wix, the copyright year can be automatically updated with Wix Velo API in 3 steps:
- Create a copyright notice with the
copyright
ID - Enable Development Mode and set up the
$w.onReady(() => { ... })
listener with the automation script - Use
$w('#copyright')
to find and get the copyright notice HTML element and update its text with the current year valuenew Date().getFullYear()
So you get something like this:
$w.onReady(() => {
const currentYear = new Date().getFullYear();
const copyrightEl = $w('#copyright');
copyrightEl.text = `Copyright © ${currentYear} allaboutweb.co`;
});
However there’s room for improvement, so don’t neglect reading this guide: Wix Copyright Footer. Add, Edit and Auto Update the Year
Dynamic Copyright Year in Shopify
By default the copyright notice year in Shopify is already configured to be automatically updated.
If it doesn’t work for you, you need to modify the copyright footer Liquid code to include the Date variable {{ 'now' | date: '%Y' }}
, like this:
<small class="copyright__content">
Copyright © 2022-{{ 'now' | date: '%Y' }}, All About Web Co. All Rights Reserved
</small>
Where ‘now’ takes the current date and date: ‘%Y’ returns only the year of this date as a text.
Detailed guide: Shopify Copyright Footer. All You Need to Know
Dynamic Copyright Year in Squarespace
There are three ways to add custom code to change the copyright year in Squarespace dynamically:
- Footer code injection
- Code block
- Embed block
The best option is to implement a copyright notice with Code Block, where you can combine CSS styles, HTML code and JS code with the current year calculation in one place.
For example:
<style>
.copyright {
text-align: center;
}
</style>
<p class="copyright">
Copyright © <span id="copyright-year">2022</span> allaboutweb.co
</p>
<script>
const currentYear = new Date().getFullYear();
const copyrightYearEl = document.getElementById("copyright-year");
if (copyrightYearEl) copyrightYearEl.textContent = currentYear;
</script>
Read more about all available options here: Squarespace Copyright Footer. Add, Edit, Auto Update the Year
Dynamic Copyright Year in Webflow
In order to dynamically change the copyright year, you need to have a paid plan that provides the code customization feature. Navigate to the site Settings, then Custom code and put the code snippet from the guide below into the Header code text area.
For example:
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', function(){
const copyrightElement = document.getElementById('copyright');
const newCopyrightText = `Copyright © ${new Date().getFullYear()} allaboutweb.co`;
if (currentYearElement) {
currentYearElement.textContent = newCopyrightText;
}
});
</script>
Read the step-by-step guide here: How to Automatically Update the Copyright Year in Webflow
Dynamic Copyright Year in Blogger (Blogspot)
To automatically update the copyright year in Blogger, remove the default copyright notice and create a new HTML/JavaScript gadget with custom HTML code with JS automation script.
For example:
<div class="copyright" style="text-align: center; font-size: 0.7em;">
Copyright © <span id="copyright-current-year"></span> allaboutweb.co
<script type="text/javascript">
let currentYearElement = document.getElementById("copyright-current-year");
if (currentYearElement) {
currentYearElement.textContent = new Date().getFullYear();
}
</script>
</div>
You can find a detailed instruction of how to add the script to you Blogger website here: Blogger Copyright Footer. Add, Edit and Auto Update the Year
Dynamic Copyright Year in Weebly
In order to change the copyright year dynamically in Weebly, you need to add the Embed code block to the site footer, then click on the Edit Custom HTML button and add copyright notice HTML code with JS script that calculates the current year.
However, a paid plan is required to be able to edit the footer block. There’s a way to bypass this limitation, you can read more about in this guide: How to Automatically Update Copyright Year on Weebly
Avoid document.write for Auto-Updating Copyright Year
You’re probably reading this article because you want to find a way to dynamically change the copyright year, and I’m pretty sure you’ve already seen some solutions over the Internet. The most popular one is a script that uses document.write
browser API.
Well, that’s not the best way to do the task. Why? Let’s ask the HTML specification.
Here is what it says:
This method has very idiosyncratic behavior. In some cases, this method can affect the state of the HTML parser while the parser is running, resulting in a DOM that does not correspond to the source of the document (e.g. if the string written is the string ). In other cases, the call can clear the current page first, as if document.open() had been called. In yet more cases, the method is simply ignored, or throws an exception. Users agents are explicitly allowed to avoid executing script elements inserted via this method. And to make matters even worse, the exact behavior of this method can in some cases be dependent on network latency, which can lead to failures that are very hard to debug. For all these reasons, use of this method is strongly discouraged.
Simply put: if you use this feature, at best auto-update won’t break anything, and at worst you might end up with bugs, errors or even crashes.
In my opinion, the potential risks are not worth the potential benefits in this particular case.
By the way, here’s an example of the code snippet I just mentioned:
<p>
©
<script>document.write(new Date().getFullYear())</script>
John Doe. All rights reserved.
</p>
Does it work? Yes!
It calculates the current year new Date().getFullYear()
and injects the result document.write(new Date().getFullYear())
directly into the HTML document.
Should you use it? It’s up to you.
At the end of the day, you might not experience any problem and you’re already aware of potential threats.
Differences Between Methods: Window load event vs. “document.write”
So, what is the difference between Window load event code snippet and the one with the document.write
method?
When we use the load event window.addEventListener('load', onLoad);
the copyright year updates as soon as the whole page has loaded. It means the old year value renders first, and right after, the year changes to the actual one. This transition happens immediately, so users most likely won’t notice it.
element.innerHTML
operates on a specific part of an HTML document, while document.write
- on the entire HTML document, it can completely replace it and bring security issues while a page is loading, which makes it hard to debug. The copyright notice is part of the footer block which appears on every page of a website. So, solving a small problem using “document.write” might become a big problem for the whole website if this API brings side effects.
Implementing a Dynamic Copyright Year for Your Unique Case
The web development landscape is diverse and all possible cases can’t be covered comprehensively in one article.
-
Consider Hiring a Software Engineer. If you’re not tech-savvy, hiring a software engineer can be a smart choice. They can tailor a solution to your specific needs and ensure a seamless update of the copyright year.
-
Exploring AI-Powered Tools: Alternatively, you can harness the power of AI tools like ChatGPT. AI can help with coding tasks, making it a viable option. When using AI, remember to be clear and specific in your requests, providing all necessary details. Here’s a prompt example:
I have a website built using Squarespace, and I’ve added a copyright notice to the footer using a Custom code block to apply custom styles. I’ll give you the code I use. Extend it to make the copyright year update automatically considering Squarespace API specifics.
{ Put your code here }
Code Examples for Auto-Updating Copyright Year in Various Frameworks
As I mentioned earlier, modern web development is based on heavy usage of different frameworks. They aim to simplify the development process. You may see it in the examples below, auto-updating logic is way more primitive than pure HTML and JavaScript.
Dynamic Copyright Year in React / NextJs / GatsbyJs
Implementation file: CopyrightNotice.tsx
import React from 'react';
const CopyrightNotice = () => {
const currentYear: number = new Date().getFullYear();
return <p>Copyright © 2022 - {currentYear} allaboutweb.co</p>;
}
export default CopyrightNotice;
Usage file: Footer.tsx
import React from 'react';
import CopyrightNotice from 'components/CopyrightNotice';
const Footer = () => {
return (
<footer>
<CopyrightNotice />
</footer>
);
}
export default Footer;
Dynamic Copyright Year in Angular
Implementation file: copyright-notice.component.ts
import { Component } from "@angular/core";
@Component({
selector: "copyright-notice",
templateUrl: "./copyright-notice.component.html",
})
export class CopyrightNotice {
currentYear = new Date().getFullYear();
}
Implementation file: copyright-notice.component.html
<p>Copyright © 2022 - {{ currentYear }} allaboutweb.co</p>
Usage file: footer.component.html
<footer>
<copyright-notice />
</footer>
Dynamic Copyright Year in Vue
Implementation file: CopyrightNotice.vue
<script>
export default {
data() {
return {
currentYear: new Date().getFullYear(),
};
},
};
</script>
<template>
<p>Copyright © 2022 - {{ currentYear }} allaboutweb.co</p>
</template>
Usage file: Footer.vue
<script>
import CopyrightNotice from "/components/CopyrightNotice.vue";
export default {
components: {
CopyrightNotice,
},
};
</script>
<template>
<footer>
<CopyrightNotice />
</footer>
</template>
Dynamic Copyright Year in Svelte
Implementation file: CopyrightNotice.svelte
<script>
const currentYear = new Date().getFullYear();
</script>
<p>Copyright © 2022 - {currentYear} allaboutweb.co</p>
Usage file: Footer.svelte
<script>
import CopyrightNotice from "./CopyrightNotice.svelte";
</script>
<footer>
<CopyrightNotice />
</footer>
Dynamic Copyright Year in Astro
Implementation file: CopyrightNotice.astro
---
const currentYear = new Date().getFullYear();
---
<p>Copyright © 2022 - {currentYear} allaboutweb.co</p>
Usage file: Footer.astro
---
import CopyrightNotice from "./CopyrightNotice.astro";
---
<footer>
<CopyrightNotice />
</footer>
Dynamic Copyright Year in PHP
Usage file:
<footer>
<p>Copyright © 2022 - <?php echo date('Y'); ?> allaboutweb.co</p>
</footer>
Dynamic Copyright Year in PHP (Wordpress)
Implementation file: functions.php
function currentYear() {
return date('Y');
}
add_shortcode('year', 'currentYear');
Usage file:
<footer>
<p>Copyright © 2022 - [year] allaboutweb.co</p>
</footer>
Summary
Automatically updated copyright year is a small part of automation that a little simplifies the website owner’s life. It’s a relatively easy task that shouldn’t take more than 10 minutes. However, there’s room for mistakes and for the sake of optimization, you may bring problems instead of benefits if you do it wrong (use of document.write).